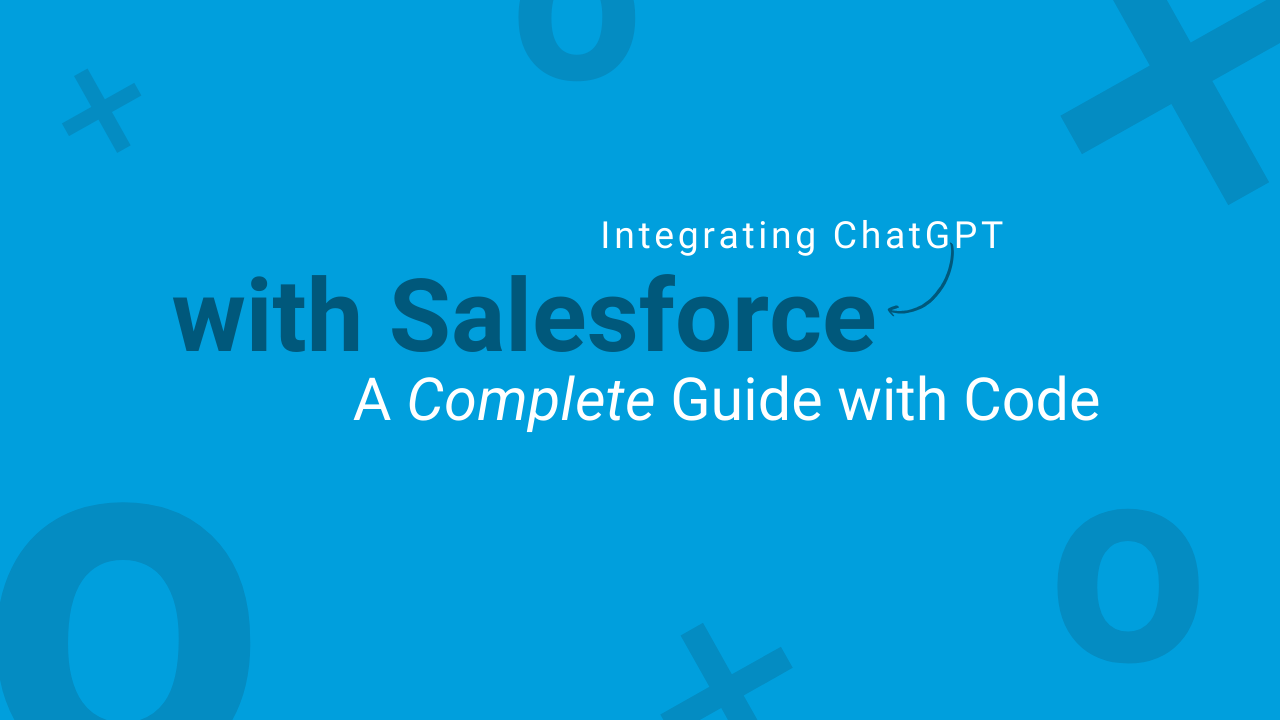
In this article, we will walk you through integrating OpenAI’s ChatGPT into Salesforce using Aura components and Lightning components. This integration will enable Salesforce users to generate responses, summaries, or even draft messages directly within the Salesforce environment, leveraging the power of ChatGPT. We will provide code templates and step-by-step instructions, making it easy for developers to implement ChatGPT in salesforce.
Table of Contents
ToggleSteps to Integrate ChatGPT with Salesforce
To get started with integrating ChatGPT in Salesforce, follow these steps:
- Set Up Remote Site Settings in Salesforce
- Create an Apex Controller to Connect with ChatGPT API
- Build the Aura Component for the Chat Interface
- Design the Lightning Component for an Enhanced UI
- Apply Custom CSS to Style the Chat Interface
- Deploy the Component and Test Your Integration
Step 1: Set Up Remote Site Settings in Salesforce
To connect Salesforce with external APIs like OpenAI’s ChatGPT, you need to configure Remote Site Settings. This ensures Salesforce can securely communicate with ChatGPT’s API.
- Navigate to Setup > Security > Remote Site Settings.
- Click New Remote Site and enter the details:
- Remote Site Name: OpenAI
- Remote Site URL: https://api.openai.com
- Ensure the site is marked as Active.
- Click Save.
Step 2: Create an Apex Controller to Connect with ChatGPT API
The Apex controller handles requests to the ChatGPT API, sending user input and receiving AI-generated responses. Below is the code template you can use.
public with sharing class ChatGPTController {
@AuraEnabled
public static String getChatGPTResponse(String userInput) {
String apiKey = 'Bearer YOUR_API_KEY'; // Replace with your OpenAI API key
Http http = new Http();
HttpRequest request = new HttpRequest();
request.setEndpoint('https://api.openai.com/v1/chat/completions');
request.setMethod('POST');
request.setHeader('Authorization', apiKey);
request.setHeader('Content-Type', 'application/json');
String requestBody = JSON.serialize(new Map<String, Object>{
'model' => 'gpt-3.5-turbo',
'messages' => new List<Map<String, Object>>{
new Map<String, Object>{
'role' => 'user',
'content' => userInput
}
},
'max_tokens' => 150
});
request.setBody(requestBody);
try {
HttpResponse response = http.send(request);
if (response.getStatusCode() == 200) {
Map<String, Object> res = (Map<String, Object>) JSON.deserializeUntyped(response.getBody());
List<Object> choices = (List<Object>) res.get('choices');
if (!choices.isEmpty()) {
Map<String, Object> choice = (Map<String, Object>) choices[0];
Map<String, Object> message = (Map<String, Object>) choice.get('message');
return (String) message.get('content');
} else {
return 'No response from ChatGPT.';
}
} else {
return 'Error: ' + response.getBody();
}
} catch (Exception e) {
return 'Exception: ' + e.getMessage();
}
}
}